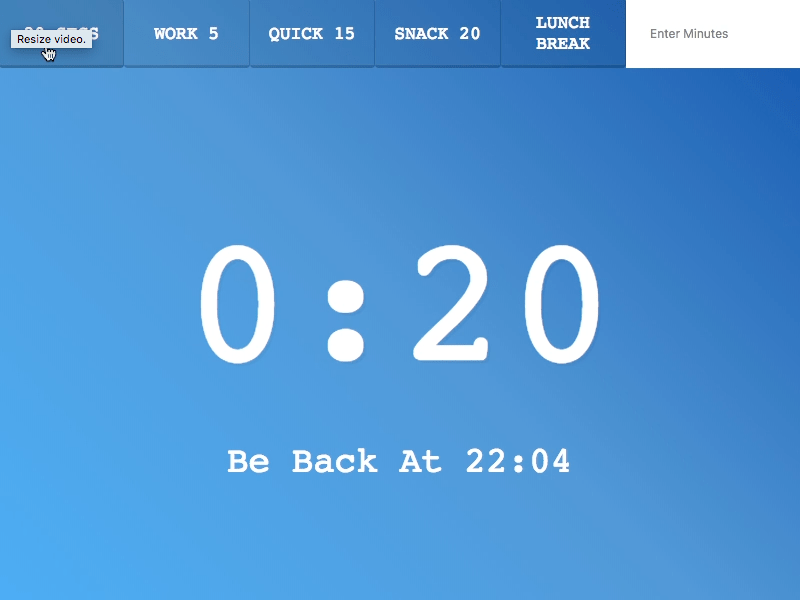
主題
製作一個倒數計時器。
步驟
Step1. 取得頁面元素並替預設
1 | const timerDisplay = document.querySelector('.display__time-left'); |
Step2. 設定計時器
1 | // 外層變數,供計時器主體使用 |
Step3. 預設的固定時間倒數按鈕
1 | // 開始計時(HTML畫面設定好的時間) |
Step4. 自訂時間倒數
1 | // HTML中的input自訂倒數時間輸入欄位監聽 |
其他
這篇練習也算是相對簡單的時間,主要是學著用變數把setInterval內容包住,
並且運用clearInterval
來避免多個計時器啟動。